Exploring JavaScript MV Frameworks Part 1 – Hello Backbonejs
JavaScript has become one of the most popular programming languages on the web. At first, developers didn’t take it seriously, simply because it was not intended for server side programming.
JavaScript has become one of the most popular programming languages on the web. At first, developers didn’t take it seriously, simply because it was not intended for server side programming.
It was a common misconception among professional developers that this language was meant for “Amateurs” as it focused only on User Interface. JavaScript got the spotlight when the usage of Ajax came to light and professional programmers gave importance to the responsiveness of the page. But now the language has become more popular than ever as the User Experience has become the key part of web development. Accessing web is not limited to browsers alone – there are lot many devices with varying screen sizes accessing the same content. With the rise of HTML5 and CSS3 the web will become more adaptive and responsive than ever and JavaScript plays a major role in it. It has also gained popularity in the server side programming which is made possible by NodeJS framework.
Increase in usage of JavaScript in modern applications demand developers to write maintainable code, separate concerns and improve testability. JavaScript is a “class” less language and it was not designed to support Object Oriented Programming, however you can achieve similar results by workarounds. So if you are a developer from an Object Oriented Programming world, then you will find it hard until you get used to it. Though there are some DOM manipulation libraries like jQuery which simplifies client side scripting of HTML, they actually do not solve the problem of effectively handling separation of concerns. You will end up writing lot many jQuery selectors and callbacks to keep the data in sync between the HTML, JavaScript and the data fetched from the server and we’re still stuck with the Spaghetti code.
Fortunately there are a few libraries and frameworks that come to rescue. Let’s explore few concepts and libraries that assist in structuring JavaScript applications!
This post is the first part of a blog series on JavaScript Frameworks and Libraries out there and I will be exploring BackboneJS here. Stay tuned for others!
What Is MV*?
Though all the frameworks out there somewhat tries to be MVC but they do not necessarily follow the pattern strictly. The idea of all the patterns is to separate Model, View and Logic that hooks the two behind which is the controller. However BackboneJS embeds the controller logic in the view itself though it efficiently maintains the separation. On the other side we do have other libraries which implement Model-View-Presenter(MVP) and Model-View-ViewModel(MVVM) pattern. For this reason we will refer these frameworks as MV* implementation.
What Is MVC?
Model – View – Controller is an architectural pattern that has been around for a long time and is widely used in server side programming. There are a few frameworks like ASP.net MVC, Ruby on Rails etc. which help web developers to easily program them.
Model – refers to application data and business rules. (a.k.a domain model, entities)
View – what user sees! (HTML page in the web browser)
Controller – mediator between the two. Manipulates the model based on the user interaction. It handles all the logic.
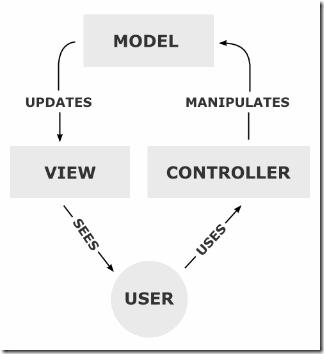
Image Source: Wikipedia
MVC In JavaScript?
Building single-page applications using JavaScript are getting popular these days and good examples of them are GMail and Google Docs. When you set out to build these type of applications you will most likely invent many of the pieces that make up an MV* coding paradigm. So instead you can make use of some of the famous libraries such as BackboneJS, KnockoutJS, AngularJS, EmberJS …. Let’s explore these frameworks in detail starting with BackboneJS.
Framework Or Just a Library?
Before you pick to work on a particular JavaScript Framework or a Library, it’s important to understand the difference between the two. Libraries just fit into your existing architecture and add a specific functionality whereas a Framework gives you an architecture and you will need to follow the rules. To make it simpler for you – Backbone and Knockout are JavaScript libraries and Ember and AngularJS are frameworks. As we explore them you will see the clear difference.
Hello Backbone
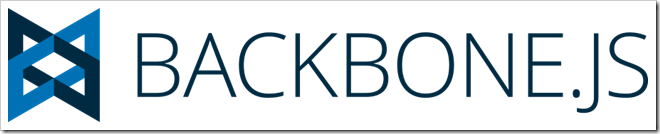
Backbone is a lightweight JavaScript library created by Jeremy Ashkenas who is also known for CoffeeScript. It is designed for supporting Single page web application and has a dependency on UnderscoreJS library which provides utility functions for common JavaScript tasks.
With Backbone, data is represented as Models, which can be created, validated, and saved to the server. Views display the model’s state and it re-renders itself when a change is triggered(via a “change” event) in the model due to an UI interaction. This way Backbone provides a structured approach of keeping the data in sync with the HTML UI.
Some of the major websites that used Backbone include USA Today, LinkedIn Mobile, Hulu, WordPress, Foursquare, Bitbucket, Khan Academy and more..
Getting started with Backbone
Script Dependency
Backbone has a dependency on UnderscoreJS or Lo-Dash for utility functions and relies on either jQuery or Zepto for DOM manipulations. So make sure you add them to your page.
<script type="text/javascript" src="../common/jquery-1.8.3.min.js"></script> <script type="text/javascript" src="../common/lodash.js"></script> <script type="text/javascript" src="js/backbone.js"></script>
Backbone.Model
$(function(){ var Person = Backbone.Model.extend({}); var person = new Person({name: "James", age: 51}); var name = person.get("name"); var age = person.get("age"); console.log(name + ":" + age); });
To create a Model class of your own, you extend Backbone.Model and pass a JavaScript object to the constructor and set the attributes. You can then easily retrieve the values from a get function. You can alternatively set the attributes using a set function.
Backbone.View
Set a template first:
<script type="text/template" id="person-template"> <div class="view"> <p>Name: <input type="text" value="<%- name%>"/></p> <p>Age: <input type="text" value="<%- age%>"/></p> </div> </script>
Set a container for rendering:
<div id="container">Your content will load in a bit..</div>
Define a view in the script:
//define view var AppView = Backbone.View.extend({ el: '#container', model: person, template: _.template($("#person-template").html()), initialize: function(){this.render();}, render: function(){this.$el.html(this.template(this.model.toJSON()));} }); // initialize view new AppView();
Views in Backbone are almost more convention than they are code so you will have to rely on some JavaScript templating library like Underscore templates or Mustache.js to do a cleaner separation of the UI. In the above example I have used Underscore’s templating solution to achieve the separation.
Your own custom Views can be created with the help of Backbone.View.extend. There are few basic properties that you must be aware of to set the View.
- el – DOM element that the view will be rendered on. In this case it is <div> element with the id “container”
- $el – a cached jQuery (or Zepto) object for the view’s element
- model – set the model data that was created using Backbone.Model
- template – Backbone is agnostic with respect to your preferred method of HTML templating. In this case Underscore’s template function is used to set the template that is defined in the “person-template”
- initialize – this function will be called by Backbone at the time of creation of the view
- render – this function is used to render the element with the view template and the data. In this case we replace the value in the “container” with the templated view that consists of data from the model
As stated in the Backbone’s documentation – the View class can also be thought of as a kind of controller dispatching events that originate from the UI, with the HTML template serving as the true view. This leads to an argument whether or not Backbone follows real MVC principles. However if you don’t learn it as a MVC library and give importance to the way it separates concerns, it should be fine.
Some time ago Backbone did have its own Backbone.Controller
, however it was renamed to Router as the naming for this component didn’t make sense from the way it was being set to use.
File Size, Download & Other useful links
File Size: 6.3Kb – minified and 56kb with Full Source and comments.
Download: Backbone website
Annotated Source: http://backbonejs.org/docs/backbone.html
Who is using it?: Check out their examples section
Stay Tuned
So that was a quick introduction to BackboneJS and an explanation on JavaScript frameworks in general. In upcoming posts you will be introduced to Ember, Knockout and Angular as well. So stay tuned!
If you have any questions write to me nish@infragistics.com or find me on twitter @nishanil
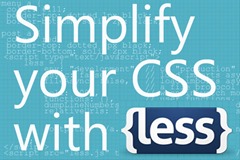
For this post, I am assuming you are a programmer like me and design is a planet just outside your universe. If you are from the design planet, you too can read on; may be there is something for you too!
Writing CSS is fun, exciting and easy until your website grows fat with pages and complicated layouts. If you have ever tried fixing a layout in such a page – you know what I mean. Did I talk about fixing a layout? Oh yeah thanks to all the browsers.
Many at times while writing CSS I wished I could write it more programmatically than just styling them. For e.g. If CSS had allowed variable declarations, I could have simply held the values in variables, perform few operations and re-use them in properties. But that’s too much to ask for from a stylesheet language which should just do styling!
Fortunately there are a few preprocessors like Sass & LESS that nicely extends CSS and adds everything a programmer ever wanted. After doing couple of research I picked up LESS to see how it works. I spent a few hours re-styling some of my demos with LESS and I am must say I am thoroughly impressed with this language. So here I’m explaining you about LESS.
So LESS
LESS is a dynamic stylesheet language that extends CSS and adds nice features like variables, mixins, operations and functions to it. More importantly it takes fewer efforts for developers to write complex CSS and build amazing looking websites real fast. LESS uses existing CSS syntax that makes learning a breeze and you can always fall back to CSS.
LESS’ first version was written in Ruby and was used as a server side language which upon compiling emitted CSS. However in the later versions, use of Ruby is deprecated and replaced by JavaScript. Adding LESS.js JavaScript file to your HTML page allows real-time compilation within browsers. It also supports server side compiling with the help of Node.js making it easier for developers to choose between the two.
Adding Less.js – Client-Side Usage
All LESS files should have the extension “.less” and you may have them under CSS directory of your webserver.
Add the following lines of code to your HTML page to ensure Pre-Compiling of CSS happens in your browser:
<link rel="stylesheet/less" type="text/css" href="css/style.less"/> <script src="js/less-1.3.3.min.js"></script>
Note: If you working on a local file system i.e. if you are accessing the page using “file:///” on Chrome or IE you may incur an error of “Cross origin requests are only supported for HTTP” or “Access Denied” respectively. These are some security related errors and I did not find a way to get rid of them. Host them on a development server and you will see this go away. However I did not find any issues with Mozilla Firefox.
Server Side Usage
If performance is what running in your mind then you can look at compiling these files on the server side. First, download and install Node.js, then using npm download the less compiler(lessc.cmd).
To compile use this command:
lessc styles.less > styles.css
For more options like CSS minifying, run lessc without parameters.
There are few nice editors out there that will let you live compile these files. For e.g. I use WebStorm which nicely compiles LESS to CSS as I type. If you are on a large project and most of your developers are comfortable on LESS then you can add the sever side compilation step to your build task.
Now that you have know how to make “.less” files and compile them to CSS, let’s look at this language in detail.
Variables
As mentioned earlier variables are one nice feature to have in stylesheets. LESS let’s you add variables with the help of @ symbol and use them into properties. Find below an example where I set background-color and color of the body with the help of variables.
@backgroundColor: #333; @color: #fff; body { background-color: @backgroundColor; color: @color; border-top: solid 10px #000; font-size: .85em; font-family: "Segoe UI", Verdana, Helvetica, Sans-Serif; margin: 0; padding: 0; }
These variables can be now re-used in the rest of the code and any change that you make to color will apply to all. CSS codes can co-exist with LESS – If you notice only two of the properties were set by variables and the rest is CSS.
Operations
Now that you know variables are a possibility in stylesheet, you must be happy to know that you can perform operations on them. It’s easy! Here’s is an example of how to do it:
@baseColor: #000; @backgroundColor: (@baseColor + #333); @color: (@backgroundColor / 3); @font-family: "Segoe UI", Verdana, Helvetica, Sans-Serif; @font-Size: 1em; #body { background-color: @backgroundColor; color: @color; border-top: solid 10px @baseColor; font-size: (@font-Size - .15em); font-family: @font-family; }
Have a look at how @backgroundColor, @color and font-size gets the calculated value from an operation. Find below the output that gets generated.
Output:
#body { background-color: #333333; color: #111111; border-top: solid 10px #000000; font-size: 0.85em; font-family: "Segoe UI", Verdana, Helvetica, Sans-Serif; }
Mixins
Mixins help you reuse the whole bunch of properties from one ruleset into another ruleset. Here’s an example:
@baseColor: #000; @font-family: "Segoe UI", Verdana, Helvetica, Sans-Serif; @font-Size: 1em; .gradients { /*local scoped variables*/ @gradientStartColor: #eaeaea; @gradientEndColor: #cccccc; background: @baseColor; /* variable from global scope */ background: linear-gradient(top, @gradientStartColor, @gradientEndColor); background: -o-linear-gradient(top, @gradientStartColor, @gradientEndColor); background: -ms-linear-gradient(top, @gradientStartColor, @gradientEndColor); background: -moz-linear-gradient(top, @gradientStartColor, @gradientEndColor); background: -webkit-linear-gradient(top, @gradientStartColor, @gradientEndColor); } #body { .gradients; border-top: solid 10px @baseColor; font-size: (@font-Size - .15em); font-family: @font-family; }
In the above code you can see how .gradients ruleset is re-used into #body. It’s a pretty nice feature, think about how less code you need to write now!
Variable Scoping
Like every other programming language, LESS provides variable scoping too. Variables are looked up locally first and when not found it will search globally. In the above example you can see that the @baseColor is being used in both .gradients and #body. If you have locally scoped variables like @gradientStartColor and @gradientEndColor they are not accessible outside the scope unless they are mixed in. In the above example #body can access those variables inside since .gradients is referred.
Check out Scope for more info.
Parametric Mixins
This is special type of ruleset which can be mixed in like classes, but accepts parameters. Here’s an example that sets up border-radius for different browsers.
.border-radius (@radius: 4px) { border-radius: @radius; -moz-border-radius: @radius; -webkit-border-radius: @radius; } #body { .gradients; .border-radius; border-top: solid 10px @baseColor; } #sidebar{ .border-radius(25px); background: #eee; }
If you look at #body it calls without parameters that’s because LESS allows to set default values of parameters which in this case is 4px. Look at #sidebar for call with parameters. You can also set multiple parameters, check this out for more information.
Functions
LESS provides few helper functions for transforming colors, string manipulation, and do math. Find below an example from the LESS documentation which uses percentage to convert 0.5 to 50%, increases the saturation of a base color by 5% and then sets the background color to one that is lightened by 25% and spun by 8 degrees:
#sidebar{ width: percentage(0.5); color: saturate(@baseColor, 5); background-color: spin(lighten(#ff0000, 25%), 8); }
Check out Function Reference for details.
Summary
By now you would have a fair idea of what LESS brings to the table. But be aware that LESS is not the only CSS preprocessor. There is Sass which stands for Syntactically Awesome Stylesheets and few others but they aren’t popular. There are various blogs out there which will tell you some good comparison between the two. I suggest you try both and stick to the syntax that you like! After all they emit CSS
Have a feedback? Find me on twitter @nishanil
