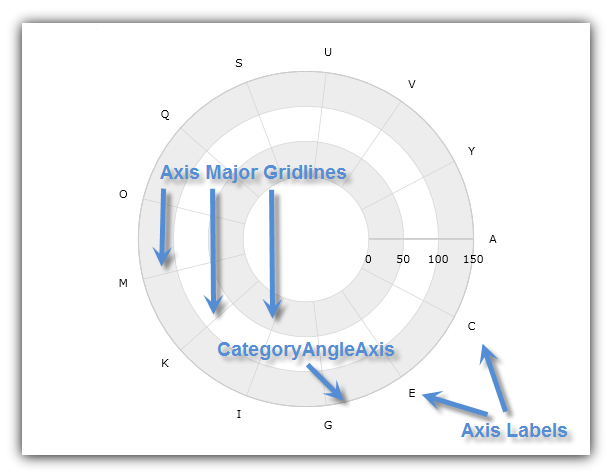
This topic demonstrates, with code examples, how to use Category Angle Axis in the UltraDataChart™ control.
The topic is organized as follows:
The CategoryAngleAxis has a shape of a circle surrounding the center of the chart with major gridlines that look like radial lines starting from center of chart and pointing outwards. (Figure 1)
Figure 1: Sample implementation of the CategoryAngleAxis shape
The CategoryAngleAxis is used only with Radial Series (in combination with NumericRadiusAxis instead of the NumericAngleAxis that is used only with Polar Series). For more information on what axis types are required by a specific series, refer to the Series Requirements topic.
The CategoryAngleAxis has the following axis crossing properties:
CrossingValue – the radius or distance from the beginning of the NumericRadiusAxis. In other words, this value determines the location of intersection of the CategoryAngleAxis on the NumericRadiusAxis. Increasing the value of the CrossingValue property, will move the CategoryAngleAxis farther from the center of the chart and decreasing it will move the angle axis closer to the center along the radius axis. For example, if the NumericRadiusAxis has a range value from 0 to 100 and a value of 50 is set on the CrossingValue property of the CategoryAngleAxis then the angle axis will cross the radius axis at value of 50. By default, the crossing value of the angle is set to the maximum value of radius axis which means that the angle axis will be rendered at the outer ring of the chart
CrossingAxis – the axis in the UltraDataChart control’s Axes collection that crosses the CategoryAngleAxis. This property must be bound to a NumericRadiusAxis, and vice-versa, if there is more than one CategoryAngleAxis and one NumericRadiusAxis in the UltraDataChart control’s Axes collection. If you don’t specify the crossing axis, then the angle axis will just assume the first axis of the NumericRadiusAxis type in the chart’s Axes collection is the correct crossing axis.
The following code snippets show how to use the CrossingAxis and CrossingValue properties of the CategoryAngleAxis in the UltraDataChart control. It sets the intersection of CategoryAngleAxis with NumericRadiusAxis at radius of 150 from the beginning of NumericRadiusAxis. The result is shown in Figure 2 below.
In Visual Basic:
Dim DataChart As New UltraDataChart() Dim categoryAngleAxis As New CategoryAngleAxis() Dim RadiusAxis As New NumericRadiusAxis() RadiusAxis.CrossingAxis = categoryAngleAxis RadiusAxis.CrossingValue = 0 categoryAngleAxis.CrossingAxis = RadiusAxis categoryAngleAxis.CrossingValue = 150 DataChart.Axes.Add(categoryAngleAxis) DataChart.Axes.Add(RadiusAxis)
In C#:
var DataChart = new UltraDataChart(); var categoryAngleAxis = new CategoryAngleAxis(); var RadiusAxis = new NumericRadiusAxis(); RadiusAxis.CrossingAxis = categoryAngleAxis; RadiusAxis.CrossingValue = 0; categoryAngleAxis.CrossingAxis = RadiusAxis; categoryAngleAxis.CrossingValue = 150; DataChart.Axes.Add(categoryAngleAxis); DataChart.Axes.Add(RadiusAxis);
Figure 2: The UltraDataChart control with CategoryAngleAxis crossing at 150 radius value of NumericRadiusAxis
The CategoryAngleAxis does not have MinimumValue and MaximumValue properties. Therefore, it always starts from the first item in data set that is bound to it and then displays axis labels and major gridlines for the rest of items in intervals equal to a value of the Interval property.
The following code snippet shows how to set interval on the CategoryAngleAxis and display every other axis label in the UltraDataChart control. The result is shown in Figure 3 below.
In Visual Basic:
Dim DataChart As New UltraDataChart() Dim categoryAngleAxis As New CategoryAngleAxis() categoryAngleAxis.Interval = 2 DataChart.Axes.Add(categoryAngleAxis)
In C#:
var DataChart = new UltraDataChart(); var categoryAngleAxis = new CategoryAngleAxis(); categoryAngleAxis.Interval = 2; DataChart.Axes.Add(categoryAngleAxis);
Figure 3: CategoryAngleAxis displaying every other axis label in the UltraDataChart
The CategoryAngleAxis always starts from the 3 o’clock position (the right-hand side of the chart). However, this can be changed by setting the CategoryAngleAxis object’s StartAngleOffset property to an angle that will offset the starting axis location in a clockwise direction. For example, a value of 90 set on the StartAngleOffset property will position the starting point for the CategoryAngleAxis at the 6 o’clock position (the bottom of the chart) and a value of 270 will start this axis at the 12 o’clock position (the top of the chart). The axis index usually increases clockwise, but you can set IsInverted to true on the CategoryAngleAxis in order to make the axis indices increase counter-clockwise instead.
The following code snippets show how to offset starting point of the CategoryAngleAxis in the UltraDataChart control by 60 degrees. The result is shown in Figure 4 below.
In Visual Basic:
Dim DataChart As New UltraDataChart() Dim categoryAngleAxis As New CategoryAngleAxis() categoryAngleAxis.StartAngleOffset = 60 DataChart.Axes.Add(categoryAngleAxis)
In C#:
var DataChart = new UltraDataChart(); var categoryAngleAxis = new CategoryAngleAxis(); categoryAngleAxis.StartAngleOffset = 60; DataChart.Axes.Add(categoryAngleAxis);
Figure 4: CategoryAngleAxis with 60 degrees offset for the starting angle value