Formatting Data Using Pipes in Ignite UI for Angular Grid
How you present data to the user is essential. Often you cannot present data as it is from the data source to the viewer.
How you present data to the user is essential. Often you cannot present data as it is from the data source to the viewer. Users need a more immersive presentation of the data. Let us consider a data source as listed below:
this.products = [ { Id: '1', Title: 'Book', ExpiryDate: new Date(), Price: 35, Rating: 3.5 }, { Id: '2', Title: 'Pen', ExpiryDate: new Date(), Price: 25, Rating: 4.0 }, { Id: '3', Title: 'Pencil', ExpiryDate: new Date(), Price: 20, Rating: 3.2 }, { Id: '4', Title: 'Bat', ExpiryDate: new Date(), Price: 135, Rating: 4.0 }, { Id: '5', Title: 'Ball', ExpiryDate: new Date(), Price: 65, Rating: 3.8 }, ];
Let’s begin by defining an instance of the igxGrid in the template and data bind the data property to the product array. It is straightforward to add Ignite UI for Angular Grid as shown in the below code listing:
<igx-grid [data]="products" [autoGenerate]="true" width="960px"> </igx-grid>
We are setting the autoGenerate property to true; do to this, Ignite UI will auto-generate all the columns by reading from the data source. The grid will be created as shown in the below image:
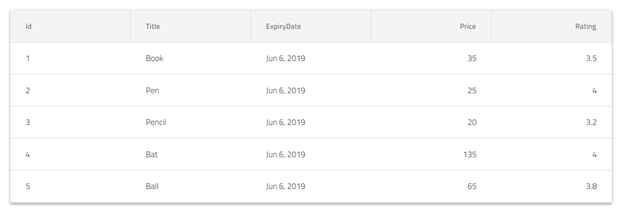
As you can see, data is displayed in a much more immersive way. Also, you may notice that Ignite UI by default has applied date pipes to the ExpiryDate column. If you are new to pipes, Angular pipes take data as input and transforms it into your desired output. The angular library provides many built-in pipes:
- UpperCasePipe
- LowerCasePipe
- CurrencyPipe
- PercentPipe
- DatePipe etc.
When you use auto generate true, Ignite UI applies DatePipe on the date object column, without it ExpiryDate will be rendered as shown in below image:

So, in autogenerate true, Ignite UI applies required pipes on the data, but still, there is some limitation of it. For example, you cannot use custom pipes or manually choose which pipe to be applied.
For better control of columns, you should configure them manually in IgxGrid. If you want to format the style or data of a particular column, for example, you have to manually configure columns in igxGrid as shown in the code listing below and then use column template.
<igx-grid [data]="products" [autoGenerate]="false" width="960px"> <igx-column field="Id" header="Id"></igx-column> <igx-column field="Title" header="Title"></igx-column> <igx-column field="ExpiryDate" header="Expiry Date"></igx-column> <igx-column field="Price" header="Price"></igx-column> <igx-column field="Rating" header="Rating"></igx-column> </igx-grid>
For a particular column, now you can configure header, column properties, and data format. Say you want to apply currency pipe to Price column, you can do that as shown in the code listing below:
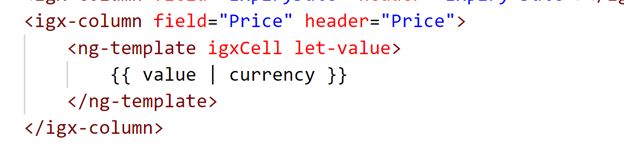
The ng-template is like an HTML template and reusable, and it replaces the content when rendered. You can use ng-template to provide
- Column template
- Header template
- Pagination template etc.
We are passing two parameters to ng-template
- ixgCell : determines that this template will be applied to a particular grid cell
- let-value : contains data value passed in the cell
Other possible input parameters for ng-template are
- igxHeader : to apply template to column header
- let-column : contains column as input data
We will talk about these in detail in another post focused on custom header template.
Now, let us modify Price and ExpiryDate columns with currency and date pipes.
<igx-grid [data]="products" [autoGenerate]="false" width="960px"> <igx-column field="Id" header="Id"></igx-column> <igx-column field="Title" header="Title"></igx-column> <igx-column field="ExpiryDate" header="Expiry Date"> <ng-template igxCell let-value> {{ value | date }} </ng-template> </igx-column> <igx-column field="Price" header="Price"> <ng-template igxCell let-value> {{ value | currency }} </ng-template> </igx-column> <igx-column field="Rating" header="Rating"></igx-column> </igx-grid>
You will get the grid rendered, as shown in the image below:
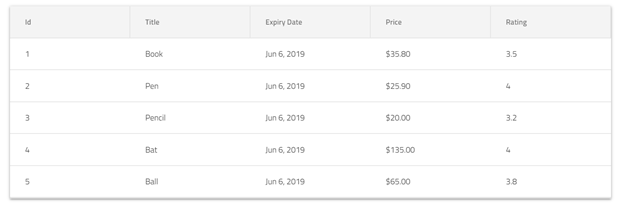
You can also pass parameters to pipes while using that in IgxGrid.
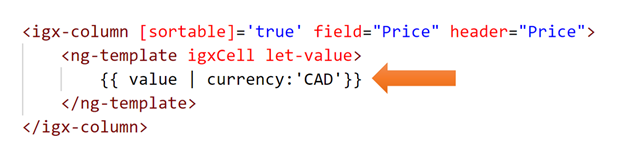
You can pass any number of parameters to pipes as supported by them, for example, additional parameters can be passed to currency pipe as shown below:
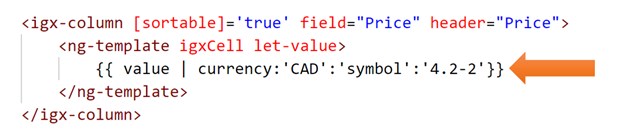
If you are working with date pipe, you can pass parameters as shown below:
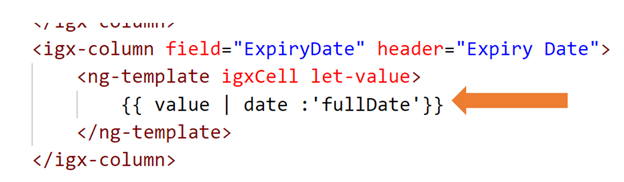
If you have created custom pipes, you can also use that, as shown below:
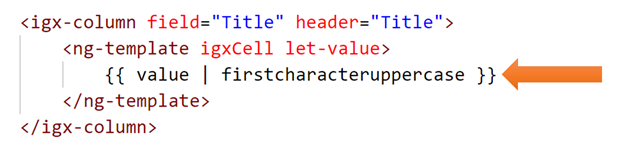
Here firstcharacteruppercase is a custom pipe. If you are not sure how to create it, learn more about it here.
Not only simple pipes, you can also use other Ignite UI for Angular components when formatting column data for better visualization. I will cover that in separate blog post. Let us put everything together to use data on igxGrid as shown below:
<igx-grid [data]="products" [autoGenerate]="false" width="960px"> <igx-column field="Id" header="Id"></igx-column> <igx-column field="Title" header="Title"> <ng-template igxCell let-value> {{ value | firstcharacteruppercase }} </ng-template> </igx-column> <igx-column field="ExpiryDate" header="Expiry Date"> <ng-template igxCell let-value> {{ value | date :'fullDate'}} </ng-template> </igx-column> <igx-column [sortable]='true' field="Price" header="Price"> <ng-template igxCell let-value> {{ value | currency:'CAD':'symbol':'4.2-2'}} </ng-template> </igx-column> <igx-column field="Rating" header="Rating"> </igx-column> </igx-grid>
Now you can see the grid is rendered as shown in the image below:
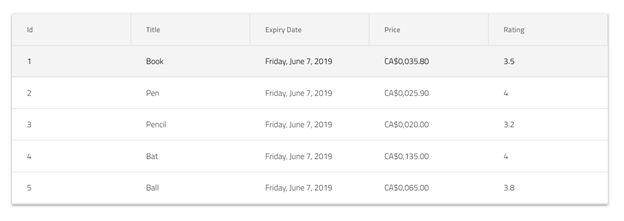
Now you may have a question that what if you are using auto-generated columns, then how you will format data in the desired way. How can you set other properties of the column such as width, sorting, paging, data format, header style, pinning, etc.? I will cover this in another blog post. As of now, I hope you find this post useful and now know how easy it is to format data using templates in Ignite UI for Angular Grid. You can learn more about Ignite UI for Angular Grid here. To learn more about pipes, watch the Desktop to Web: Transforming Data with Angular Pipes video