Simplifying Angular Data Binding to .NET Developers
Handling Angular data binding may be time consuming and sometimes even too complex. This blog post will show you how to simplify the process effectively.
At my job, I get the opportunity to talk to many .NET developers who want to learn Angular. Often, I’ve seen that they bring their .NET skills and work to map that in the learning of Angular. While the effort and drive to learn is there Angular is not .NET.
Since Angular is a pure JavaScript library, I’ll simplify basic but important concepts of Angular to .NET developers in this post series.
In this article, we’ll learn about Data Bindings in Angular. Luckily, Data Binding in Angular is much simpler than in .NET.
First, Let’s revise some of data binding techniques in .NET. For example, in ASP.NET MVC, you do data binding using a model. View is bound
- To an object
- To a complex object
- To a collection of objects
Essentially, in ASP.NET MVC, you do data binding to a model class. On the other hand, in WPF, you have data binding modes available. You can set the mode of data binding in XAML, as follows:
- One-way data binding
- Two-way data binding
- One-time data binding
- One-way to source data binding
If you are following MVVM patterns, then you might be using INotifyPropertyChanged interface to achieve two-way data binding. Therefore, there are many ways data bindings are achieved in world of .NET.
Data binding in Angular, however, is much simpler.
If you are extremely new in Angular, then let me introduce you to Components. In Angular applications, what you see in the browser (or elsewhere) is a component. A component consists of the following parts:
- A TypeScript class called Component class
- A HTML file called Template of the component
- An optional CSS file for the styling of the component
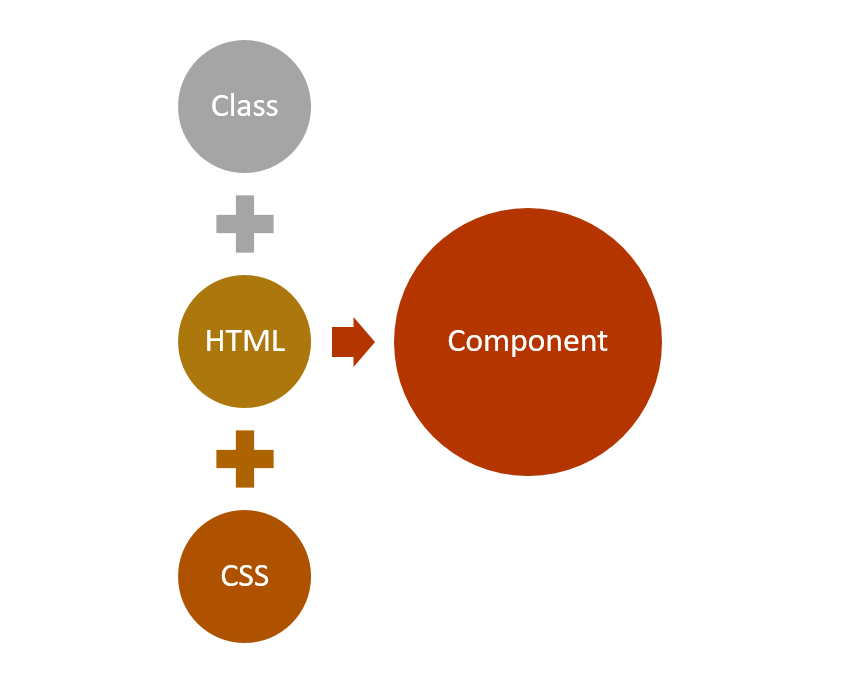
In Angular, Data Binding determines how data will flow in between Component class and Component Template.
Angular provides us three types of data bindings. They are as follows:
- Interpolation
- Property Binding
- Event Binding
Let’s see each one by one.
Interpolation
Angular interpolation is one-way data binding. It is used to pass data from component class to the template. The syntax of interpolation is {{propertyname}}.
Let’s say that we have component class as shown below:
export class AppComponent { product = { title: 'Cricket Bat', price: 500 }; }
We need to pass the product from the component class to the template. Keep in mind that to keep example simple, I’m hard coding the value of the product object, however, in a real scenario, data could be fetched from the database using the API. We can display value of the product object using interpolation, as shown in the listing below:
<h1>Product</h1> <h2>Title : {{product.title}}</h2> <h2>Price : {{product.price}}</h2>
Using interpolation, data is passed from the component class to the template. Ideally, whenever the value of the product object is changed, the template will be updated with the updated value of the product object.
In Angular, there is something called ChangeDetector Service, which makes sure that value of property in the component class and the template are in sync with each other.
Therefore, if you want to display data in Angular, you should use interpolation data binding.
Property Binding
Angular provides you with a second type of binding called “Property Binding”. The syntax of property binding is the square bracket []. It allows to set the property of HTML elements on atemplate with the property from the component class.
So, let’s say that you have a component class like the one below:
export class AppComponent { btnHeight = 100; btnWidth = 100; }
Now, you can set height and width properties of a button on template with the properties of the component class using the property binding.
<button [style.height.px] = 'btnHeight' [style.width.px] = 'btnWidth' > Add Product </button >
Angular Property Binding is used to set the property of HTML Elements with the properties of the component class. You can also set properties of other HTML elements like image, list, table, etc. Whenever the property’s value in the component class changes, the HTML element property will be updated in the property binding.
Event Binding
Angular provides you third type of binding to capture events raised on template in a component class. For instance, there’s a button on the component template and, on click of the button, you want to call a function in component class. You can do this using Event Binding. The syntax behind Event Binding is (eventname).
For this example, you might have a component class like this:
export class AppComponent { addProduct() { console.log('add product'); } }
You want to call addProduct function on the click of the button on the template. You can do this using event binding:
<h1>Product</h1> <button (click)='addProduct()'> Add Product </button>
You can do event binding with all events of a HTML elements which is part of Angular ngZone. You can learn more about it here.
Angular provides you these three bindings. In event binding, data flows from template to class and, in property binding and interpolation, data flows from class to template.
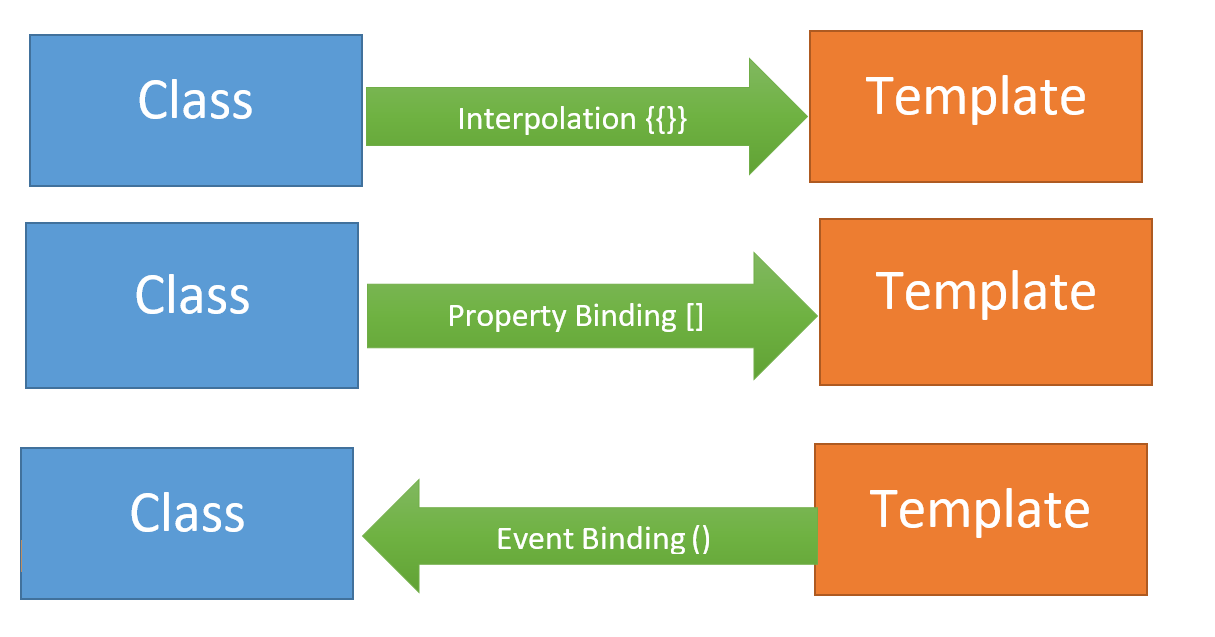
Two-Way Data Binding
Angular does not have built-in two-way data binding, however, by combining Property Binding and Event Binding, you can achieve Two-Way Data Binding.
Angular provides us a directive, ngModel, to achieve two-way data binding, and It’s very easy to use. First, import FormsModule and then you can create two-way data binding:
export class AppComponent { name = 'foo'; }
We can two-way data bind the name property with an input box:
<input type="text" [(ngModel)]='name' /> <h2>{{name}}</h2>
As you see, we are using [(ngModel)] to create two-way data binding in between input control and name property. Whenever a user changes the value of the input box, the name property will be updated and vice versa.
As a .NET developer, now you might have realized that data binding in Angular is much simpler, and all you need to know is four syntaxes. I hope you find this post useful and, in further posts, we will cover other topics of Angular.
If you like this post, please share it. Also, if you have not checked out Infragistics Ignite UI for Angular Components, be sure to do so! They have 30+ material based Angular components to help you code web apps faster.