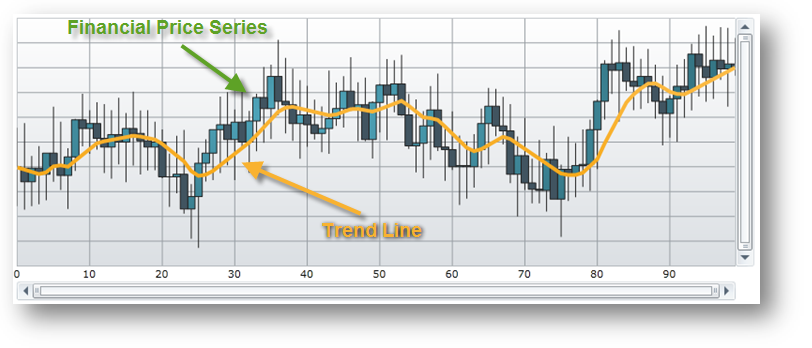
This topic explains, with code examples, how to use the trend lines in the XamDataChart™ control.
In the XamDataChart control, trend line is a line that helps identify a trend in data bound to a Series through its ItemsSource property.
Figure 1 – Sample implementation of trend line in the Data Chart.
Most types of series provide support for plotting trend lines in the chart control and these series are listed in the following table.
Table 1 – Series that support trend lines
The Data Chart supports the following types of trend lines through Series object’s TrendLineType property.
Table 2 – Types of trend lines.
All properties of trend lines start with the "TrendLine" string and they are provided by each Series object.
Table 3 – Trend lines properties.
This section provides code examples for using trend lines only with the Financial Price Series. However, the same logic can be applied to other series that support trend lines by replacing the FinancialPriceSeries object with other type of series.
This code snippet demonstrates how to show the Weighted Moving Average trend line on the Financial Price Series using its TrendLineType property. Assigning different TrendLineType enumeration value to this property will change which trend line is displayed with the series.
In C#:
// create financial price series with a trend line
var series = new FinancialPriceSeries();
// ...
series.TrendLineType = TrendLineType.WeightedAverage;
series.TrendLinePeriod = 10;
// add a series to the chart
DataChart.Series.Add(series);
Figure 2 – Financial Price Series with Weighted Moving Average trend line in the Chart control.
This code snippet demonstrates how to style a trend line.
In C#:
// create financial price series with and style its trend line
var series = new FinancialPriceSeries();
// ...
series.TrendLineBrush = new SolidColorBrush(Colors.Orange);
series.TrendLineThickness = 5.0;
// add a series to the chart
DataChart.Series.Add(series);
Figure 3 – Financial Price Series with styled trend line in the Data Chart.